AngularHuskyPrettier . 5 min read
Angular + Prettier + Husky
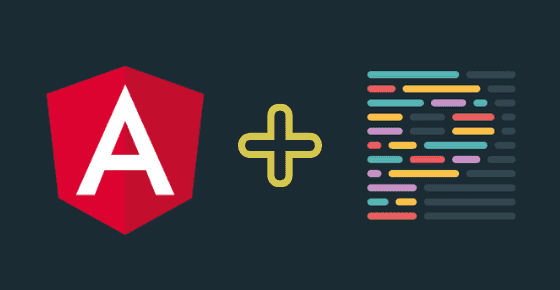
What is Prettier?
Prettier is an opinionated code formatter. It is a great way to keep code formatted consistently for you and your team.
What is husky?
Husky describes itself as “Git hooks made easy”. It provides pre-commit and pre-push hooks.
Pre-push/Pre-commit hooks are nothing but commands which you would want to run every time you push/commit something.
In this tutorial, we are going to configure husky and prettier in an Angular application to standardize and automatize the code format.
Step 1: Create an Angular project using CLI
ng new angularApp
Step 2: Install prettier
npm install --save-dev prettier
Step 3: Configure prettier
On the root of the project, create a file called .prettierrc with these prettier options,
{
"bracketSpacing": true,
"semi": false,
"singleQuote": true,
"trailingComma": "es5",
"printWidth": 80
}
Create another file .prettierignore at the root of the project
package.json
package-lock.json
yarn.lock
dist
Step 4: TSLint + Prettier
In this step let’s add tslint-config-prettier so that it disables all conflicting rules that may cause such problems and both could be used without any problem. Prettier takes care of the formatting whereas tslint takes care of all the other things.
npm install --save-dev tslint-config-prettier
Then, extend your tslint.json, and make sure tslint-config-prettier is at the end:
"extends": [
"tslint:recommended",
"tslint-config-prettier"
],
Step 5: Install pretty-quick
We want prettier to only run on our changed files, instead of running on whole project. pretty-quick does exactly that.
npm install --save-dev pretty-quick
Step 5: Install husky
This is the most important step, where we create git hooks. First, install husky,
npm install husky --save-dev
Pre hooks are very useful and could be used in protecting the quality of your code at a much, much higher level. Pre hooks run a shell command which you specify and if the command fails with exit status 1, the process will get terminated. So imagine that you won’t be able to push the code if your tests are failing or your ts files are not properly linted. No broken builds 🙂
"husky": {
"hooks": {
"pre-commit": "pretty-quick --staged && ng lint && ng test",
"pre-push": "ng build --aot true"
}
}
Here we are doing three things in pre-commit hook,
- pretty-quick –staged – This will run prettier to format staged files and those files will be re-staged automatically after formatting
- ng lint – It will run the lint command, to check lint errors and warnings
- ng test – This will run the unit test cases of project
In pre-push hook, we are creating the build of the project. The best thing is if any of the tasks fail it will terminate the process. This will prevent us from lint errors, fail test cases, and broken builds.
You can find all the source code in this repository Github.
Hey!
Need help with software development or extending your team? You’re in the right place.
Let’s make cool things happen 🚀